In some projects, you might see components organized into their own directories. This means each component has a folder with all its related files. While this might seem like overkill at first, there are good reasons to think about structuring your components this way. Let's explore why this can be helpful.
Looking at a Sample Application
Consider the example application and Storybook setup shown below:
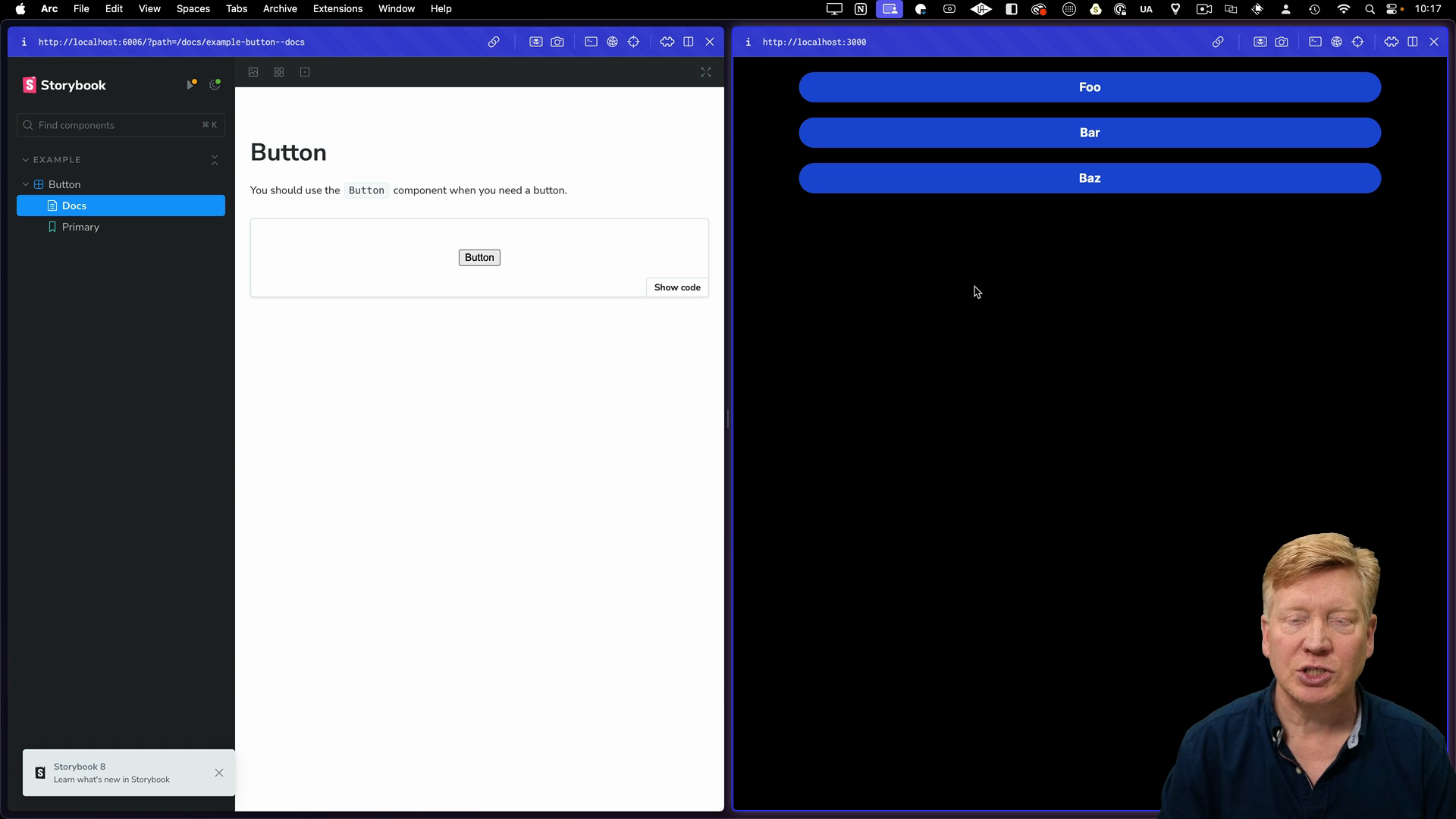
On the right side of our development environment, we have the app using the Button
component. On the left side, we have Storybook, which shows the button by itself for development and testing.
In Storybook, we have three main sections for the Button
component, some tests, and the Stories for the button. Let's look at how that that maps to the project's file structure.
Understanding the File Structure
Inside of the project directory is a __tests__
directory, which contians a file Button.test.tsx
. We can run these tests with pnpm test
in the terminal.
To run Storybook and see the button by itself, we use pnpm storybook
. The button stories are in the src/stories
directory. Here, we find two files: Button.stories.mdx
and Button.stories.ts
.
Then inside of the app
directory, the button component has two more files: Button.tsx
for the component code and Button.module.css
for the styles.
In total, our simple Button
component involves five different files: the component code, its CSS styles, the Storybook stories, the documentation, and the tests.
This can get messy, especially when changing the button, since we need to find and update files in different places.
Putting Components into Directories
To fix this, we can make a new directory called Button
at the top level app
directory in our project. We'll then move all five button files into this new directory. This way, everything about the Button
component is in one place:
// inside `src/app/Button`
Button.test.tsx
Button.stories.mdx
Button.stories.ts
Button.tsx
Button.module.css
Challenge
Your challenge now is to make sure that the import when using the Button
component remains ./Button
, even after moving the files into the Button
directory.
Try to solve this on your own, then check out the next lesson to see how I did it.