Picking up from where we left off, we'll start by moving the Button.test.tsx
, Button.stories.tsx
, and Button.tsx
files into the app/Button
directory. This way, all the files related to the Button
component are in one place.
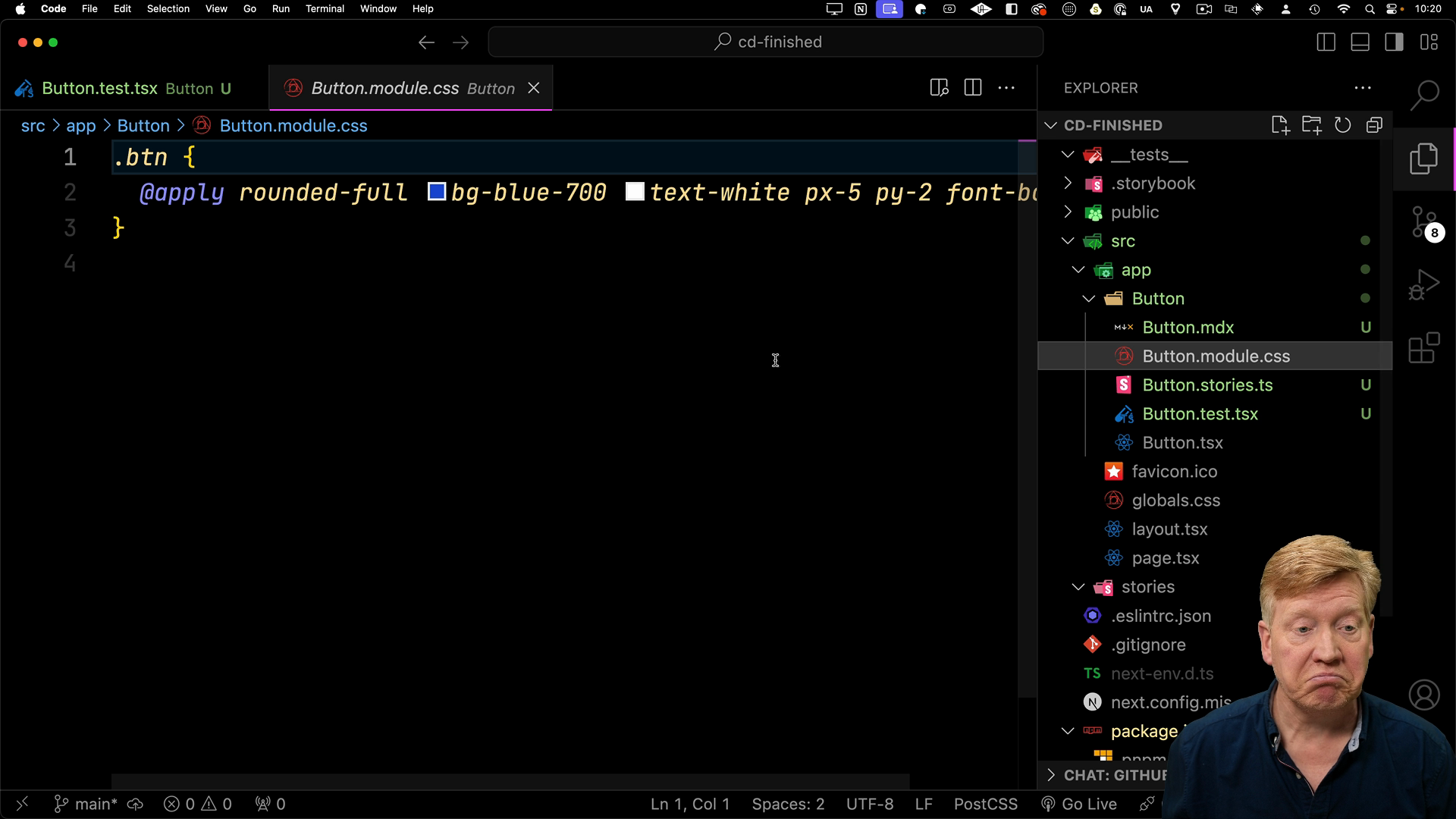
Updating Import Paths
However, when opening page.tsx
we can see that the import has been updated to have Button
listed twice:
// inside page.tsx
import { Button } from './Button/Button';
The goal is to simplify it to:
import { Button } from './Button'
In order to do this, we can create an index.ts
file inside of the Button
directory. Inside the file, we'll add an export:
// inside `src/app/Button/index.ts`
export { Button } from './Button';
Because the component has its own imports, we can make edits like changing CSS colors and the changes will reflect in the application.
This approach is also nice because we can search for Button
and find the source file directly, rather than having to navigate through an index
file first.
Handling Default Exports
Sometimes, you might have a component defined as a default export. For example, your Button.tsx
file might look like this:
export default function Button() { ... }
In this case, you would need to adjust the index.tsx
file slightly to export the default
:
export { default } from './Button'
Advantages of Directory Structure
Organizing components into directories like this helps make the project structure easier to understand. The directory is also easier to share and reuse across the application or in other projects.
While it might seem like a lot for smaller components, using this structure from the beginning sets a good foundation and encourages good organization.